Commit a82b05e
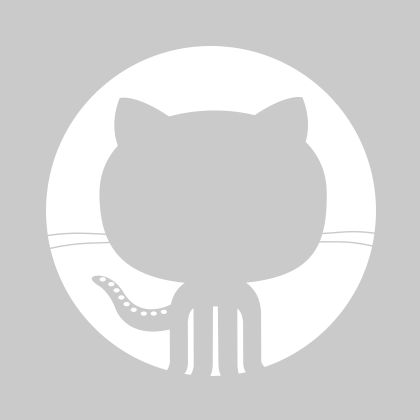
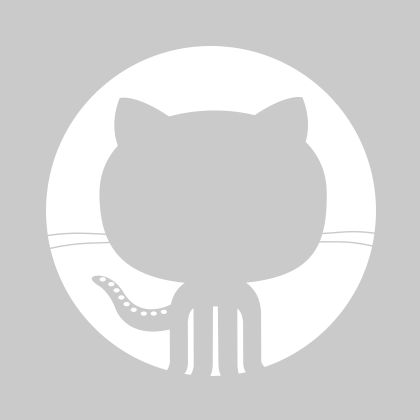
Keijiro Takahashi
Keijiro Takahashi
1 parent 761c098 commit a82b05e
File tree
8 files changed
+208
-137
lines changed- Assets
- CubeCluster
- Shader
- Material
- Particle System
8 files changed
+208
-137
lines changedLines changed: 65 additions & 26 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
6 | 6 |
| |
7 | 7 |
| |
8 | 8 |
| |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
9 | 21 |
| |
10 | 22 |
| |
11 | 23 |
| |
| |||
21 | 33 |
| |
22 | 34 |
| |
23 | 35 |
| |
24 |
| - | |
25 |
| - | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
26 | 39 |
| |
| 40 | + | |
27 | 41 |
| |
28 | 42 |
| |
29 |
| - | |
30 |
| - | |
31 |
| - | |
32 |
| - | |
33 |
| - | |
34 |
| - | |
35 |
| - | |
36 |
| - | |
37 |
| - | |
38 |
| - | |
39 |
| - | |
40 |
| - | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
41 | 60 |
| |
42 | 61 |
| |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
43 | 66 |
| |
44 | 67 |
| |
45 | 68 |
| |
| |||
52 | 75 |
| |
53 | 76 |
| |
54 | 77 |
| |
55 |
| - | |
| 78 | + | |
56 | 79 |
| |
57 |
| - | |
58 |
| - | |
59 |
| - | |
60 |
| - | |
61 |
| - | |
62 |
| - | |
| 80 | + | |
63 | 81 |
| |
64 | 82 |
| |
65 | 83 |
| |
66 | 84 |
| |
67 |
| - | |
68 |
| - | |
69 |
| - | |
| 85 | + | |
| 86 | + | |
70 | 87 |
| |
| 88 | + | |
| 89 | + | |
| 90 | + | |
71 | 91 |
| |
72 | 92 |
| |
73 |
| - | |
74 |
| - | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
| 107 | + | |
| 108 | + | |
| 109 | + | |
75 | 110 |
| |
76 | 111 |
| |
77 | 112 |
| |
78 | 113 |
| |
79 | 114 |
| |
| 115 | + | |
| 116 | + | |
80 | 117 |
| |
| 118 | + | |
| 119 | + | |
81 | 120 |
| |
82 | 121 |
|
Lines changed: 26 additions & 21 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
26 | 26 |
| |
27 | 27 |
| |
28 | 28 |
| |
29 |
| - | |
30 |
| - | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
31 | 32 |
| |
32 |
| - | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
33 | 38 |
| |
34 | 39 |
| |
35 | 40 |
| |
| |||
85 | 90 |
| |
86 | 91 |
| |
87 | 92 |
| |
88 |
| - | |
89 |
| - | |
90 |
| - | |
91 |
| - | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
92 | 97 |
| |
93 | 98 |
| |
94 |
| - | |
95 |
| - | |
| 99 | + | |
| 100 | + | |
96 | 101 |
| |
97 | 102 |
| |
98 | 103 |
| |
99 | 104 |
| |
100 | 105 |
| |
101 | 106 |
| |
102 | 107 |
| |
103 |
| - | |
104 |
| - | |
| 108 | + | |
| 109 | + | |
105 | 110 |
| |
106 | 111 |
| |
107 | 112 |
| |
108 |
| - | |
109 |
| - | |
| 113 | + | |
| 114 | + | |
110 | 115 |
| |
111 | 116 |
| |
112 | 117 |
| |
113 |
| - | |
| 118 | + | |
114 | 119 |
| |
115 | 120 |
| |
116 | 121 |
| |
117 | 122 |
| |
118 | 123 |
| |
119 |
| - | |
| 124 | + | |
120 | 125 |
| |
121 |
| - | |
| 126 | + | |
122 | 127 |
| |
123 | 128 |
| |
124 | 129 |
| |
125 |
| - | |
| 130 | + | |
126 | 131 |
| |
127 | 132 |
| |
128 | 133 |
| |
129 | 134 |
| |
130 |
| - | |
| 135 | + | |
131 | 136 |
| |
132 |
| - | |
| 137 | + | |
133 | 138 |
| |
134 | 139 |
| |
135 | 140 |
| |
136 |
| - | |
| 141 | + | |
137 | 142 |
| |
138 |
| - | |
| 143 | + | |
139 | 144 |
| |
140 | 145 |
| |
141 | 146 |
| |
|
Lines changed: 29 additions & 55 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
111 | 111 |
| |
112 | 112 |
| |
113 | 113 |
| |
114 |
| - | |
| 114 | + | |
115 | 115 |
| |
116 | 116 |
| |
117 | 117 |
| |
| |||
146 | 146 |
| |
147 | 147 |
| |
148 | 148 |
| |
149 |
| - | |
| 149 | + | |
150 | 150 |
| |
151 | 151 |
| |
152 | 152 |
| |
| |||
498 | 498 |
| |
499 | 499 |
| |
500 | 500 |
| |
| 501 | + | |
501 | 502 |
| |
502 | 503 |
| |
503 | 504 |
| |
| |||
516 | 517 |
| |
517 | 518 |
| |
518 | 519 |
| |
| 520 | + | |
| 521 | + | |
519 | 522 |
| |
520 | 523 |
| |
521 | 524 |
| |
| |||
529 | 532 |
| |
530 | 533 |
| |
531 | 534 |
| |
532 |
| - | |
| 535 | + | |
533 | 536 |
| |
534 | 537 |
| |
535 |
| - | |
| 538 | + | |
| 539 | + | |
536 | 540 |
| |
537 |
| - | |
| 541 | + | |
| 542 | + | |
| 543 | + | |
| 544 | + | |
| 545 | + | |
| 546 | + | |
| 547 | + | |
| 548 | + | |
| 549 | + | |
| 550 | + | |
| 551 | + | |
| 552 | + | |
| 553 | + | |
| 554 | + | |
| 555 | + | |
| 556 | + | |
| 557 | + | |
| 558 | + | |
538 | 559 |
| |
539 | 560 |
| |
540 | 561 |
| |
| |||
815 | 836 |
| |
816 | 837 |
| |
817 | 838 |
| |
818 |
| - | |
| 839 | + | |
819 | 840 |
| |
820 | 841 |
| |
821 |
| - | |
| 842 | + | |
822 | 843 |
| |
823 |
| - | |
| 844 | + | |
824 | 845 |
| |
825 | 846 |
| |
826 | 847 |
| |
| |||
1034 | 1055 |
| |
1035 | 1056 |
| |
1036 | 1057 |
| |
1037 |
| - | |
1038 |
| - | |
1039 |
| - | |
1040 |
| - | |
1041 |
| - | |
1042 |
| - | |
1043 |
| - | |
1044 |
| - | |
1045 |
| - | |
1046 |
| - | |
1047 |
| - | |
1048 |
| - | |
1049 |
| - | |
1050 |
| - | |
1051 |
| - | |
1052 |
| - | |
1053 |
| - | |
1054 |
| - | |
1055 |
| - | |
1056 |
| - | |
1057 |
| - | |
1058 |
| - | |
1059 |
| - | |
1060 |
| - | |
1061 |
| - | |
1062 |
| - | |
1063 |
| - | |
1064 |
| - | |
1065 |
| - | |
1066 |
| - | |
1067 |
| - | |
1068 |
| - | |
1069 |
| - | |
1070 |
| - | |
1071 |
| - | |
1072 |
| - | |
1073 |
| - | |
1074 |
| - | |
1075 |
| - | |
1076 |
| - | |
1077 |
| - | |
1078 |
| - | |
1079 |
| - | |
1080 |
| - | |
1081 |
| - | |
1082 |
| - | |
1083 |
| - |
Lines changed: 5 additions & 5 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
101 | 101 |
| |
102 | 102 |
| |
103 | 103 |
| |
104 |
| - | |
105 |
| - | |
106 |
| - | |
107 |
| - | |
108 |
| - | |
| 104 | + | |
109 | 105 |
| |
110 | 106 |
| |
111 | 107 |
| |
| |||
114 | 110 |
| |
115 | 111 |
| |
116 | 112 |
| |
| 113 | + | |
| 114 | + | |
| 115 | + | |
| 116 | + | |
117 | 117 |
| |
118 | 118 |
| |
119 | 119 |
| |
|
0 commit comments