|
1 |
| -# [Clean Architecture](https://blog.cleancoder.com/uncle-bob/2012/08/13/the-clean-architecture.html) Flutter Project |
| 1 | +--- |
| 2 | +description: >- |
| 3 | + This is the implementation of clean architecture by Uncle Bob. We can |
| 4 | + implement this project to make applications with many modules and components |
| 5 | + also make sure application can long maintenance. |
| 6 | +--- |
2 | 7 |
|
| 8 | +# Introduction |
3 | 9 |
|
4 |
| -<p align="center"> |
5 |
| -<img alt="last commit" src="https://img.shields.io/github/last-commit/imamabdulazis/FlutterCleanArchitecture/main"/> |
6 |
| -<img alt="last commit" src="https://app.codacy.com/project/badge/Grade/bc288b78ed0a4377b5e5ef72fdbad535"/> |
7 |
| -<img alt="issue" src="https://img.shields.io/github/issues/imamabdulazis/FlutterCleanArchitecture?color=critical"/> |
8 |
| -<img alt="last commit" src="https://img.shields.io/github/repo-size/imamabdulazis/FlutterCleanArchitecture?color=orange"/> |
9 |
| -<img alt="last commit" src="https://img.shields.io/github/search/imamabdulazis/FlutterCleanArchitecture/flutter%20clean%20architecture?color=brightgreen"/> |
10 |
| -<img alt="last commit" src="https://img.shields.io/github/downloads/imamabdulazis/FlutterCleanArchitecture/total"/> |
11 |
| -</p> |
| 10 | +## 1.0.1 - 2021-11-03 |
12 | 11 |
|
13 |
| -<p>This is implementation clean architecture by Uncle Bob. We can implementation this project to make application with many module and component. |
14 |
| -First we must prepare some library for supporting our project like injector, api consume like dio and many other library we must intall it.<br/> |
15 |
| -In this case I made a facebook clone with rest API and you can clone it anytime and feel free to wait for my update app to make sure the app works properly. |
16 |
| -</p> |
| 12 | +### Fixed |
17 | 13 |
|
18 |
| -## Getting Started. |
| 14 | +* Update user interface with new SDK flutter 2.\* |
19 | 15 |
|
20 |
| -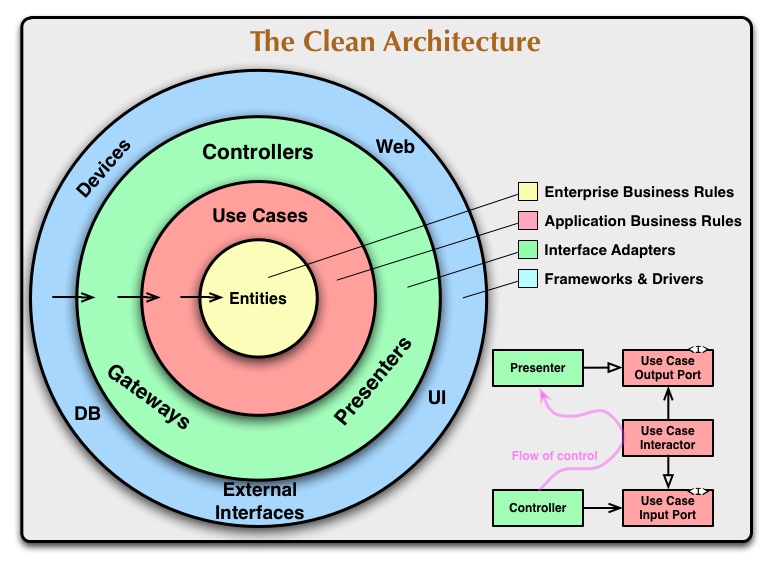 |
| 16 | +### Changed |
21 | 17 |
|
| 18 | +* Translation from arb to JSON file. |
22 | 19 |
|
23 |
| -> ### Installing |
24 |
| -You can clone this library using : |
| 20 | +## 1.0.2 - 2021-11-20 |
25 | 21 |
|
26 |
| -```shell |
27 |
| -git clone https://github.com/imamabdulazis/Flutter-Clean-Architectur-Bloc-RxDart-Dio.git |
28 |
| -``` |
| 22 | +### Added |
29 | 23 |
|
30 |
| -NOTE : |
31 |
| -This project is using flutter version ```1.22.3``` and channel ```stable``` |
32 |
| -> ⚠️ If you are get any problem to this project please feel free to create issue |
| 24 | +* Introduced new design documentation about clean architecture flutter project. |
33 | 25 |
|
34 |
| -<br/> |
35 | 26 |
|
36 |
| -> ### Library Requirement |
37 |
| -<p> |
38 |
| -We need some library to make our apps is more simple code and clean. |
39 |
| -This is some library and you can click to install from pub dev. |
40 |
| -</p> |
41 | 27 |
|
42 |
| - |
43 |
| -| Library | Usability | Star | |
44 |
| -| :-----------------------------------------------------------------|:--------------------------|:---------:| |
45 |
| -| [dartz](https://pub.dev/packages/dartz) | Data Handling | [](https://github.com/spebbe/dartz) | |
46 |
| -| [dio](https://pub.dev/packages/dio) | Http client | [](https://github.com/felangel/bloc) | |
47 |
| -| [equatable](https://pub.dev/packages/equatable) | Getting data abstract | [](https://github.com/felangel/equatable) | |
48 |
| -| [flutter_hooks](https://pub.dev/packages/flutter_hooks) | More simple code stateful | [](https://github.com/rrousselGit/flutter_hooks) | |
49 |
| -| [flutter_bloc](https://pub.dev/packages/flutter_bloc) | State management | [](https://github.com/felangel/bloc) | |
50 |
| -| [get_it](https://pub.dev/packages/get_it) | Component Injector | [](https://github.com/fluttercommunity/get_it) | |
51 |
| -| [getx](https://pub.dev/packages/get) | Component handling | [](https://github.com/jonataslaw/getx) | |
52 |
| -| [pedantic](https://pub.dev/packages/pedantic) | Code formatting | [](https://github.com/google/pedantic) | |
53 |
| -| [rxdart](https://pub.dev/packages/rxdart) | Data handling async | [](https://github.com/ReactiveX/rxdart) | |
54 |
| -| [logger](https://pub.dev/packages/logger) | Beautiful terminal log | [](https://github.com/google/pedantic) | |
55 |
| -| [shared_preferences](https://pub.dev/packages/shared_preferences) | Save data local storage | [](https://github.com/flutter/plugins) | |
56 |
| - |
57 |
| ->##Usecase |
58 |
| -<p> |
59 |
| -This is part more easy to handling some data error from network or other. |
60 |
| -</p> |
61 |
| - |
62 |
| -```dart |
63 |
| -abstract class UseCase<Type, Params> { |
64 |
| - Stream<Either<Failure, Type>> build(Params params); |
65 |
| -
|
66 |
| - Stream<Either<Failure, Type>> execute(Params params) { |
67 |
| - return build(params).onErrorResume((error) { |
68 |
| - print("error from streams : $error"); |
69 |
| - Failure failure; |
70 |
| -
|
71 |
| - if (error is Failure) { |
72 |
| - failure = error; |
73 |
| - } else if (error is DioError) { |
74 |
| - failure = ServerFailure(message: error.message); |
75 |
| - } else { |
76 |
| - failure = AnotherFailure(message: "$error"); |
77 |
| - } |
78 |
| -
|
79 |
| - return Stream.value(Left(failure)); |
80 |
| - }); |
81 |
| - } |
82 |
| -} |
83 |
| -
|
84 |
| -class NoParams extends Equatable { |
85 |
| - @override |
86 |
| - List<Object> get props => []; |
87 |
| -} |
88 |
| -``` |
89 |
| - |
90 |
| -<br/> |
91 |
| -<br/> |
92 |
| - |
93 |
| ->##Datasource Option |
94 |
| -<p>In this part we are enable to make option where data come from local or network</p> |
95 |
| -
|
96 |
| ->####Base Datasource (local or network or other) |
97 |
| -```dart |
98 |
| -abstract class BaseDataSourceFactory<T> { |
99 |
| - T createData(DataSourceState dataSourceState); |
100 |
| -} |
101 |
| -
|
102 |
| -enum DataSourceState { network, local } |
103 |
| -``` |
104 |
| - |
105 |
| ->####Datasource factory |
106 |
| -```dart |
107 |
| -class BindingDataSourceFactory |
108 |
| - extends BaseDataSourceFactory<BindingDataSource> { |
109 |
| - BindingRemote _bindingRemote; |
110 |
| - BindingLocal _bindingLocal; |
111 |
| -
|
112 |
| - BindingDataSourceFactory({ |
113 |
| - @required BindingRemote bindingRemote, |
114 |
| - @required BindingLocal bindingLocal, |
115 |
| - }) : _bindingRemote = bindingRemote, |
116 |
| - _bindingLocal = bindingLocal; |
117 |
| -
|
118 |
| - @override |
119 |
| - BindingDataSource createData(DataSourceState dataSourceState) { |
120 |
| - switch (dataSourceState) { |
121 |
| - case DataSourceState.network: |
122 |
| - return _bindingRemote; |
123 |
| - break; |
124 |
| - case DataSourceState.local: |
125 |
| - return _bindingLocal; |
126 |
| - default: |
127 |
| - throw UnsupportedError( |
128 |
| - 'DataSourceState $dataSourceState is not applicable in BindingDataSourceFactory'); |
129 |
| - } |
130 |
| - } |
131 |
| -} |
132 |
| -``` |
133 |
| - |
134 |
| -<br/> |
135 |
| - |
136 |
| ->##Resource String Component |
137 |
| ->####Handling component handling (i18n or Localization) |
138 |
| -<p> |
139 |
| -In this part we must handling component string and prepare if our project |
140 |
| -is using multiple language |
141 |
| -</p> |
142 |
| - |
143 |
| -```dart |
144 |
| -//NOTE : base translation class |
145 |
| -abstract class Translation { |
146 |
| - String get msgEmailInUse; |
147 |
| - String get msgInvalidCredentials; |
148 |
| - String get msgInvalidField; |
149 |
| - String get msgRequiredField; |
150 |
| - String get msgUnexpectedError; |
151 |
| -
|
152 |
| - String get addAccount; |
153 |
| - String get confirmPassword; |
154 |
| - String get email; |
155 |
| - String get enter; |
156 |
| - String get login; |
157 |
| - String get name; |
158 |
| - String get password; |
159 |
| - String get reload; |
160 |
| - String get wait; |
161 |
| -} |
162 |
| -
|
163 |
| -//NOTE : implement to language class |
164 |
| -
|
165 |
| -class ID implements Translation { |
166 |
| - String get msgEmailInUse => 'Email sudah digunakan'; |
167 |
| - String get msgInvalidCredentials => 'Username atau password salah.'; |
168 |
| - String get msgInvalidField => 'Bidang tidak valid'; |
169 |
| - String get msgRequiredField => 'Kolom yang harus diisi'; |
170 |
| - String get msgUnexpectedError => 'Ada yang salah. Silahkan coba lagi nanti.'; |
171 |
| -
|
172 |
| - String get addAccount => 'Buat sebuah akun'; |
173 |
| - String get confirmPassword => 'Konfirmasi sandi'; |
174 |
| - String get email => 'Email'; |
175 |
| - String get enter => 'Gabung'; |
176 |
| - String get login => 'Login'; |
177 |
| - String get name => 'Nama'; |
178 |
| - String get password => 'Kata sandi'; |
179 |
| - String get reload => 'Muat ulang'; |
180 |
| - String get wait => 'Tunggu...'; |
181 |
| -} |
182 |
| -
|
183 |
| -
|
184 |
| -class R { |
185 |
| - static Translation string = ID(); |
186 |
| -
|
187 |
| - static void load(Locale locale) { |
188 |
| - switch (locale.toString()) { |
189 |
| - default: |
190 |
| - string = ID(); |
191 |
| - break; |
192 |
| - } |
193 |
| - } |
194 |
| -} |
195 |
| -
|
196 |
| -example calling : |
197 |
| - R.string.msgEmailInUse |
198 |
| -output : |
199 |
| - Email sudah digunakan |
200 |
| -
|
201 |
| -``` |
202 |
| - |
203 |
| - |
204 |
| - |
205 |
| -<br/> |
206 |
| -<br/> |
207 |
| - |
208 |
| -> ### Credit |
209 |
| -
|
210 |
| -This architecture is made with love:blush: and more things using great tutorials by great people, please visit this |
211 |
| -project credit, thank you. |
212 |
| - |
213 |
| -[Flutter Community](https://flutter.dev/community)<br/> |
214 |
| -[Filled stack](https://www.filledstacks.com)<br/> |
215 |
| -[Reso Coder](https://resocoder.com)<br/> |
216 |
| -[Rezky Aulia Pratama](https://github.com/rezkyauliapratama)<br/> |
217 |
| -[Ashish Rawat](https://dev.to/ashishrawat2911/handling-network-calls-and-exceptions-in-flutter-54me) |
0 commit comments